Introduction
Lightning Web Components (LWC) offer seamless communication between parent and child components, making it easy to share data and invoke methods.
In this blog post, we’ll create one more practical example to explore how to establish a parent-child relationship in LWC using the @api decorator.Â
If you’re not familiar with the @api decorator in Salesforce LWC, I recommend checking out the post linked below. It provides a detailed explanation of the @api decorator with an example. Once you’ve gone through it, come back to this post for further insights.
What Will You Learn?
- How to pass data from a parent component to a child component.
- How to call a child component’s method from the parent component.
- The role of the @api decorator in enabling this communication.
Scenario Overview
We’ll create two LWC components:
1. Parent Component: This will allow the user to:
- Set a counter value through an input field.
- Increment the counter by 100 using a button (Button Name = Add 100 to counter)
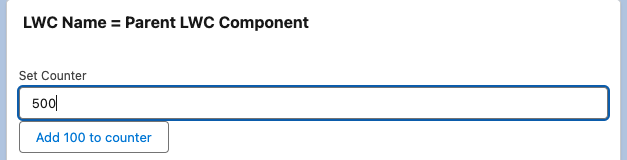
2. Child Component: This will:
- Display the counter value received from the parent.
- Increment the counter when the parent calls its method. (That is when Add 100 to counter button is clicked on Parent Component)

This is how the LWC Component will look
The Code
Parent Component
The parent component facilitates user interaction and communicates with the child component.
parentComponent.html
parentComponent.js
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
startCounter = 0;
// Capture user input
handleStartChange(event) {
this.startCounter = parseInt(event.target.value); // Convert input to a number
}
// Call the child component's method
handleMaximizeCounter() {
console.log("handleMaximizeCounter is called");
this.template.querySelector('c-child-component').maximizeCounter();
}
}
Child Component
The child component receives data from the parent and exposes a method to modify the data.
childComponent.html
Count Value: {counter}
childComponent.js
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
@api counter = 0; // Expose counter property to parent
// Expose method to parent to increment counter
@api maximizeCounter() {
console.log("maximizeCounter is called");
this.counter += 100; // Increment counter
}
}
How Does It Work?
1. Passing Data to the Child Component:
- The parent component passes the ‘startCounter’ value to the child component using the counter property.
- The @api decorator in the child component ensures that the counter property is exposed as a public property for data binding.
2. Calling a Child Method from the Parent:
- When the user clicks the “Add 100 to counter” button, the parent calls the ‘maximizeCounter’ method in the child component.
- The @api decorator allows the parent to access this method.
3. Dynamic Updates:
- When the ‘maximizeCounter’ method is invoked, the counter value in the child component updates, and the new value is displayed dynamically.
Code Explanation
Parent Component
1. Input Field and Button:
- The input field allows the user to set a starting value for the counter.
- The button triggers the ‘handleMaximizeCounter’ method.
2. Communication with Child:
- The ‘startCounter’ value is passed to the child via the counter attribute.
- The parent uses the querySelector method to call the ‘maximizeCounter’ method in the child.
Child Component
1. Counter Property:
- The counter property is declared with @api, allowing the parent to pass data to it.
2. Exposed Method:
- The ‘maximizeCounter’ method is exposed to the parent, allowing it to increment the counter value.
Output Example
1. Initially:
- The input field in the parent is empty, and the child displays Count Value: 0.
2. After Setting a Value:
- Enter 200 in the parent’s input field. The child updates to display Count Value: 200.
3. After Clicking the Button:
- Click the “Add 100 to counter” button. The child updates to display Count Value: 300.
Key Points
1. @api Decorator:
- Essential for exposing properties and methods in the child component to the parent component.
2. Dynamic Interaction:
- Updates in the parent component reflect immediately in the child component.
3. Component Reusability:
- By separating the logic into two components, you enhance modularity and reusability.
Conclusion
This example demonstrates how the @api decorator in LWC facilitates effective communication between parent and child components.
By leveraging this relationship, you can build modular, scalable, and dynamic components in Salesforce.
Understanding these concepts will empower you to create robust Lightning Web Components for a variety of use cases.
For more details, check out the official documentation: LWC @api Decorator Reference