Introduction
Oracle SQL is a powerful and widely-used tool for managing and querying relational databases, making it a key skill for many tech roles.
This post dives into essential Oracle SQL interview questions, ranging from intermediate to advanced levels.
These questions are designed to challenge your understanding of complex queries, optimization techniques, and advanced database functionalities, helping you prepare for technical interviews with confidence.
Whether you’re aiming to deepen your expertise or tackle tough interview scenarios, this guide has you covered!
Differentiate between the WHERE clause and the HAVING clause in Oracle SQL?
In Oracle SQL, both the WHERE clause and the HAVING clause are used to filter data, but they serve different purposes and are applied at different stages of query execution.
Here’s a detailed differentiation:
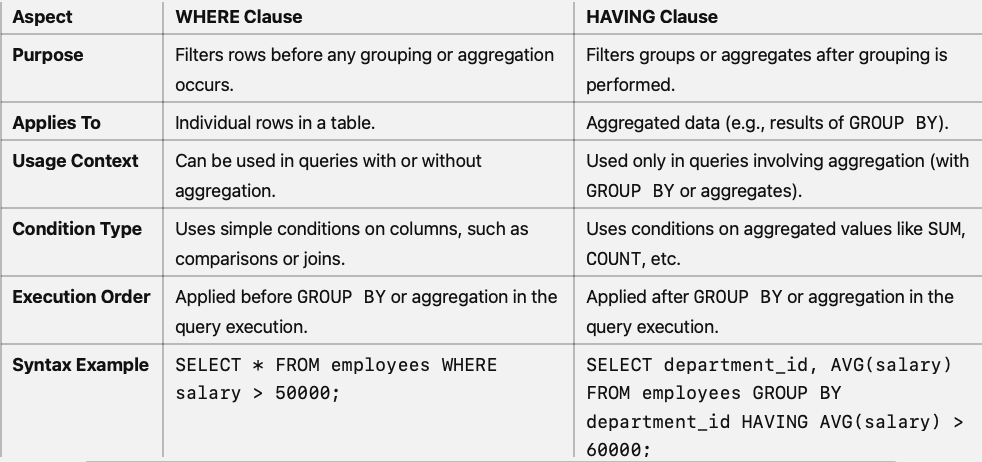
Key Notes:
1.WHERE Clause:
Use WHERE to filter individual rows based on specific criteria.
- Does not work with aggregate functions directly.
SELECT employee_id, department_id, salary
FROM employees
WHERE salary > 50000;
This filters employees with a salary greater than 50,000.
2.HAVING Clause:
Use HAVING to filter aggregated results.
- Often used in conjunction with GROUP BY.
SELECT department_id, AVG(salary) AS avg_salary
FROM employees
GROUP BY department_id
HAVING AVG(salary) > 60000;
This calculates the average salary for each department and filters departments where the average salary exceeds 60,000.
Explain the difference between RANK and DENSE_RANK functions in Oracle SQL?
The RANK and DENSE_RANK functions in Oracle SQL are both analytical functions used to assign ranks to rows based on the order of a specific column or expression. However, they differ in how they handle ties (rows with the same rank).
Here’s a detailed comparison:
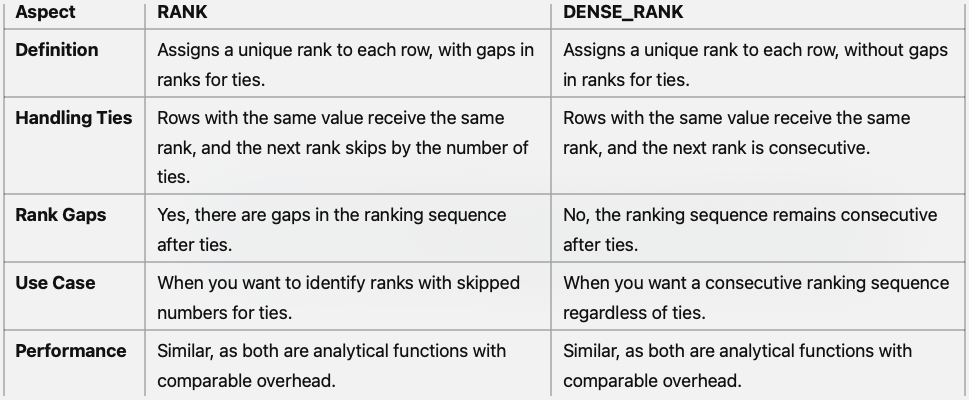
Syntax
RANK() OVER ([PARTITION BY column] ORDER BY column ASC/DESC)
DENSE_RANK() OVER ([PARTITION BY column] ORDER BY column ASC/DESC)
What are some advantages of using bind variables in Oracle SQL?
Bind variables in Oracle SQL offer several advantages:
- Improved Performance: Reduces hard parsing by reusing execution plans for queries with different values.
- Reduced SQL Injection Risk: Safeguards against SQL injection attacks by separating query logic from data values.
- Memory Efficiency: Minimizes the use of the shared pool by reusing SQL statements.
- Scalability: Enhances system scalability by handling multiple queries efficiently.
- Faster Execution: Speeds up query execution by avoiding repeated parsing and optimizing shared cursor usage.
- Flexibility: Allows dynamic input values without modifying query structure.
Differentiate between VARCHAR and VARCHAR2 data types?
In Oracle, VARCHAR and VARCHAR2 are used to store variable-length character data, but there are key differences:
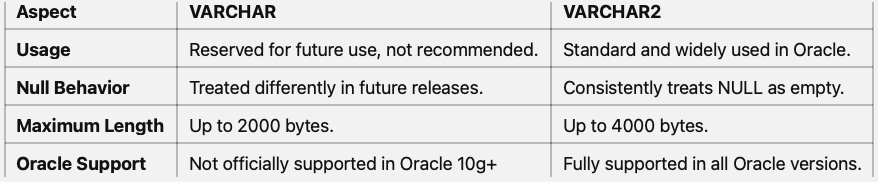
Recommendation: Always use VARCHAR2, as it is reliable and optimized for Oracle.
Explain the difference between a correlated subquery and a nested subquery
The key differences between a correlated subquery and a nested subquery are:
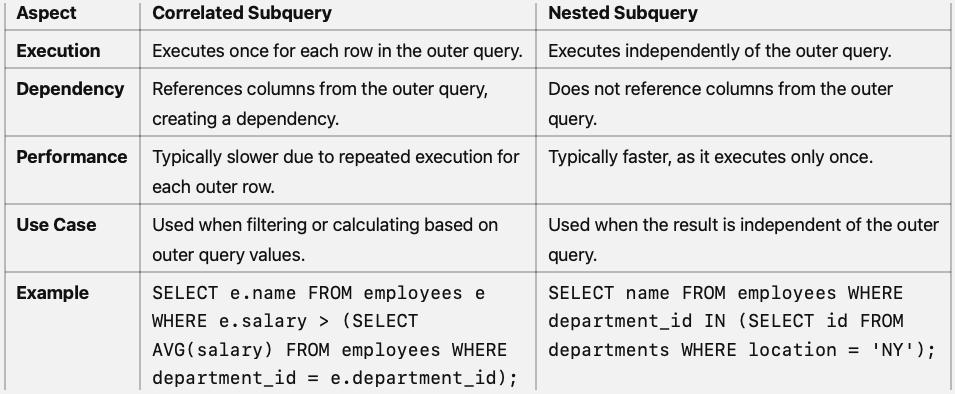
Summary:
- Correlated Subquery: Dependent on the outer query; row-by-row evaluation.
- Nested Subquery: Independent of the outer query; evaluated once.
Explain the concept of materialized views and their benefits
Materialized views are pre-computed copies of database queries stored as tables.
They improve query performance by providing pre-aggregated or filtered data, reducing processing needs for repeated queries.
Materialized views can be refreshed on-demand, automatically, or incrementally (fast refresh), depending on requirements.
Benefits
- Improved Query Performance:
Speeds up complex queries by storing precomputed results. - Reduced Computation:
Offloads computation from frequently run, resource-intensive queries. - Data Aggregation:
Efficiently stores aggregated data (e.g., sums, averages) for reporting purposes. - Disconnection from Base Tables:
Can be refreshed periodically, making it useful for scenarios where live data is not required. - Partitioning and Indexing:
Allows indexing and partitioning on the materialized view to enhance query efficiency further. - Replication:
Useful in distributed databases for data replication and synchronization.
- Improved Query Performance:
Use Cases:
- Reporting and analytics.
- Data warehousing.
- Query optimization for frequently accessed data.
Explain the difference between UNION and UNION ALL in SQL.
The main differences between UNION and UNION ALL in SQL are:
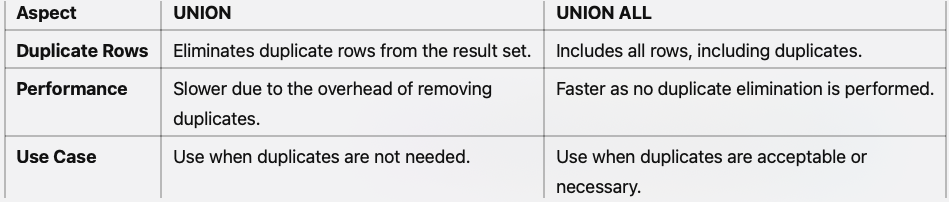
Example:
Using UNION:
Combines results from both tables and removes duplicates
SELECT name FROM table1
UNION
SELECT name FROM table2;
Using UNION ALL:
Combines results from both tables and includes all rows (duplicates retained).
SELECT name FROM table1
UNION ALL
SELECT name FROM table2;
What is the use of MERGE statements in SQL?
The MERGE statement in SQL is used to combine INSERT, UPDATE, and optionally DELETE operations into a single query.
It is commonly used for upsert operations (update if the record exists, insert if it does not).
Use Cases:
- Synchronize Tables: Update or insert data from one table into another.
- Data Warehousing: Maintain fact and dimension tables by merging new data.
- Performance Optimization: Reduces the need for separate INSERT and UPDATE statements.
MERGE INTO target_table t
USING source_table s
ON (t.key = s.key)
WHEN MATCHED THEN
UPDATE SET t.column1 = s.column1
WHEN NOT MATCHED THEN
INSERT (column1, column2) VALUES (s.column1, s.column2);
Benefits:
- Simplifies complex operations.
- Improves performance by reducing multiple SQL statements into one.
- Ensures atomicity for combined INSERT and UPDATE.
What is the use of execution plan?
An execution plan is the sequence of operations that the database performs to run a SQL statement.
It’s used to:
- Optimize Queries: Identify inefficiencies like full table scans or missing indexes.
- Understand Query Behavior: See how SQL statements are processed.
- Improve Performance: Refine queries or adjust database structures for better execution.
How would you view the execution plan?
1. In Oracle SQL Developer:
- Write your query.
- Click on the Explain Plan button or press F10.
2.Using SQL Query:
EXPLAIN PLAN FOR
SELECT * FROM employees;
SELECT * FROM TABLE (DBMS_XPLAN.DISPLAY());
3. Using Autotrace:
SET AUTOTRACE ON;
SELECT * FROM employees;
Key Insights from Execution Plan:
- Operation type (e.g., Full Table Scan, Index Scan).
- Cost of operations.
- Cardinality (estimated rows).
- Execution order and dependencies.
What do you mean by ACID properties?
The ACID properties ensure reliable database transactions and maintain data integrity. They stand for:
1.Atomicity:
•Ensures that a transaction is treated as a single, indivisible unit.
•Either all operations within the transaction are completed, or none are applied.
2.Consistency:
•Guarantees that a transaction brings the database from one valid state to another.
•Ensures data integrity rules are maintained.
3.Isolation:
•Ensures that transactions are executed independently, without interference.
•Prevents intermediate states of one transaction from being visible to others.
4.Durability:
•Ensures that once a transaction is committed, the changes are permanent, even in case of system failure.
Importance
ACID properties are fundamental for maintaining database reliability, ensuring correct and predictable results for all transactions.
Conclusion
Mastering Oracle SQL is a crucial skill for database professionals, and understanding essential interview questions can give you a competitive edge in job interviews.
By familiarizing yourself with core concepts like joins, subqueries, indexes, optimization techniques, and advanced features like materialized views and analytical functions, you can confidently tackle a wide range of questions.
Keep practicing, exploring, and refining your SQL expertise to excel in your Oracle SQL journey.
Best of luck with your interview 🚀