Introduction
In Salesforce Lightning Web Components (LWC), decorators are part of ECMA script which are used to add some extra functionality to your functions or methods.
Among these, the @api decorator is crucial for enabling component communication and data sharing.
This blog post explains the @api decorator, its significance, and practical applications, with examples for clarity.
If you’re unfamiliar with Decorators, I recommend checking out the posts below to learn about the different types of LWC decorators in Salesforce.
What is the @api decorator?
- The @api decorator in LWC is used to expose a public property or expose the method of a child component to its parent component.
- @api is always used in Javascript file of child component, so that the properties or methods present in child LWC component can be exposed to parent LWC component.
- As a result – parent LWC component can access and interact with child LWC component’s property or method.
It enables the following:
- Enables communication and data sharing from Parent LWC component to Child LWC component.
- Exposing Public Properties: Allows parent components to pass data to the child component.
- Exposing Public Methods: Permits parent components to call methods defined in the child component.
The @api decorator ensures that the marked properties and methods of a LWC component are accessible from outside of that component.
When to use @api Decorator?
@api decorator is used to:
1. Communicate Between Parent and Child Components:
Use @api to facilitate seamless data flow from parent to child component.
2. Creating Reusable Components:
When building reusable components, @api allows the parent to configure the child component dynamically.
3. Simplifying Component Interaction:
Parent components can trigger specific logic in child components using @api-exposed methods.
Syntax and Usage
To declare a public property or method and expose it to the parent LWC component, use the @api decorator. This ensures that the particular property or method is accessible from the parent component.
Here’s an example of a Child LWC Component’s JavaScript file:
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
@api publicProperty; // Public property exposed to the parent LWC component
@api publicMethod() // Public property exposed to the parent LWC component
{
console.log('This method is accessible from the parent component');
}
}
Example 1 : Exposing Child Component's Properties with @api
We have learnt that – @api decorator is always used in the Child LWC Component’s JavaScript file.
In this example, we declare @api in Child Component’s property so that Parent component can access the Child Component’s property (which was declared with @api) and pass a value to it.
Lets say that the Child LWC Component name is – childComponent and Parent LWC Component name is – parentComponent
Requirement:
Create a property in the Child LWC Component and set its value to ‘I love Salesforce’.
Then, access the same Child LWC Component from the Parent LWC Component and update that property’s value to ‘I love Writtee.com’.
Child Component
Child Component : childComponent.html
{itemName}
Explanation
{itemName}:
- •This is a dynamic binding in LWC. It displays the current value of the itemName property.
- •Initially, itemName is set to “I love Salesforce” (defined in the JavaScript file).
Child Component : childComponent.js
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
@api itemName = "I love Salesforce" // by declaring @api, we are exposing itemName property as public property.
// Now, we will update the value of the itemName property directly from the parent component.
}
Explanation
@api Decorator:
- Marks the ‘itemName’ property as public.
- Makes itemName accessible from the parent component. The parent can update this property dynamically.
itemName Property:
- Initially set to “I love Salesforce”.
- The parent component can override or update this value.
Parent Component
Parent Component : parentComponent.html
Explanation
 <c-child-component> :
- This tag refers to the child component (childComponent).
- The item-name=”I love Writtee.com” attribute is used to update the itemName property of the child component.
- The parent sets the itemName value to “I love Writtee.com”, which overrides the default value defined in the child component.
Parent Component : parentComponent.js
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
}
Explanation
- No additional logic is required in the JavaScript file for this specific example. The relationship between the parent and child is handled in the parent HTML file.
Output:
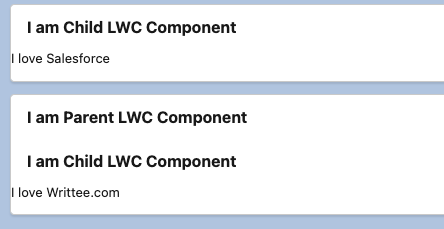
Explanation: How the above Code Works Together
- Child Component Initialization:
•The itemName property in the child component is initially set to “I love Salesforce”.
- Parent Overrides Child’s Property:
•The parent component passes a value (“I love Writtee.com”) to the child component using the item-name attribute.
•Since the itemName property in the child component is marked with @api, the parent can dynamically override its value.
Rendered Output:
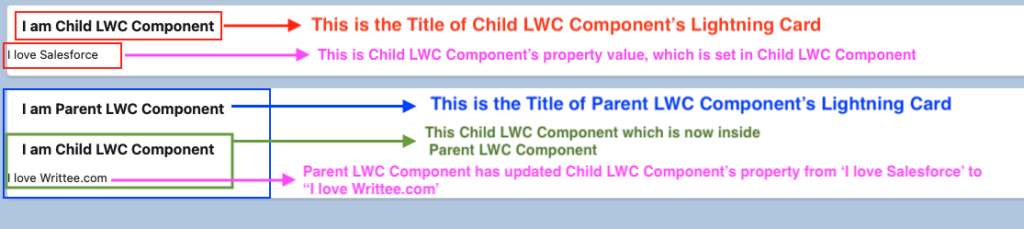
Example 2: Exposing Child Component's Methods with @api
In this example, we declare @api in the javascript file of Child Component’s method so that Parent component can access and trigger the Child Component’s method.
Requirement:
Create a property in the Child LWC Component and set its value to ‘I love Salesforce’.
Then, add a button in the Parent LWC Component.Â
When a user clicks the button on Parent LWC Component, the Child Component’s property value should update from ‘I love Salesforce’ to ‘I love my country’.
(Until and unless the button on Parent LWC Component is clicked, the Child LWC Component’s property value should not update)
Child Component
Child Component : childComponent.html
{itemName}
Explanation
{itemName}:
- This is a dynamic binding in LWC that displays the value of the itemName property on the UI.
Child Component : childComponent.js
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
itemName = "I love Salesforce"
@api handleChangeValue(){
this.itemName = "I love my country"
}
}
Explanation
itemName:
- A property initialized with the value “I love Salesforce”. It is displayed in the child component’s UI.
@api handleChangeValue():
- This is a public method that allows the parent component to modify the itemName property in the child component.
- When this method is called, the value of itemName is updated to “I love my country”.
- The @api decorator ensures this method is accessible from the parent component.
Parent Component
Parent Component : parentComponent.html
Explanation
<c-child-component>:
- This represents the child component (childComponent).
- The parent component uses this tag to include the child component in its UI.
lightning-button:
- A button with the label “Submit”.
- When clicked, the handleClick method in the parent component’s JavaScript file is triggered.
Parent Component : parentComponent.js
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
handleClick(){
this.template.querySelector("c-child-component").handleChangeValue();
}
}
Explanation
handleClick():
- This method is triggered when the button in the parent component is clicked.
- It uses this.template.querySelector() to locate the child component.
- The handleChangeValue() method of the child component is then called, which updates the itemName property in the child component.
Before clicking the button
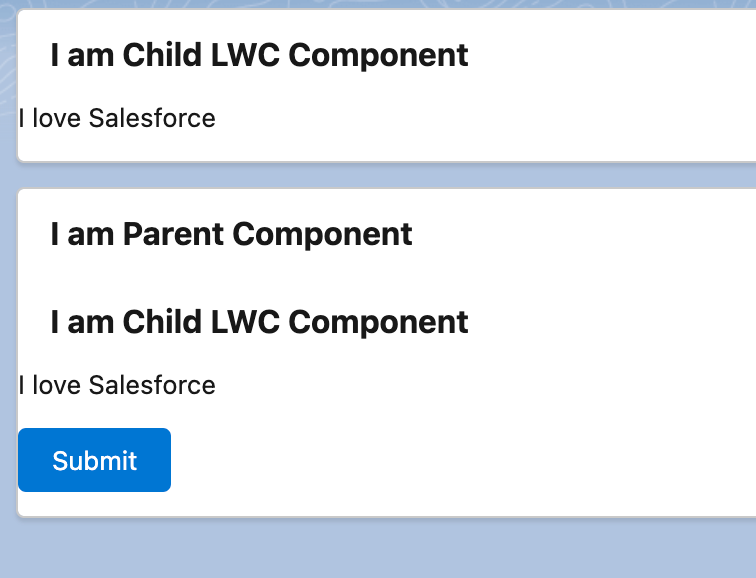
After clicking the button
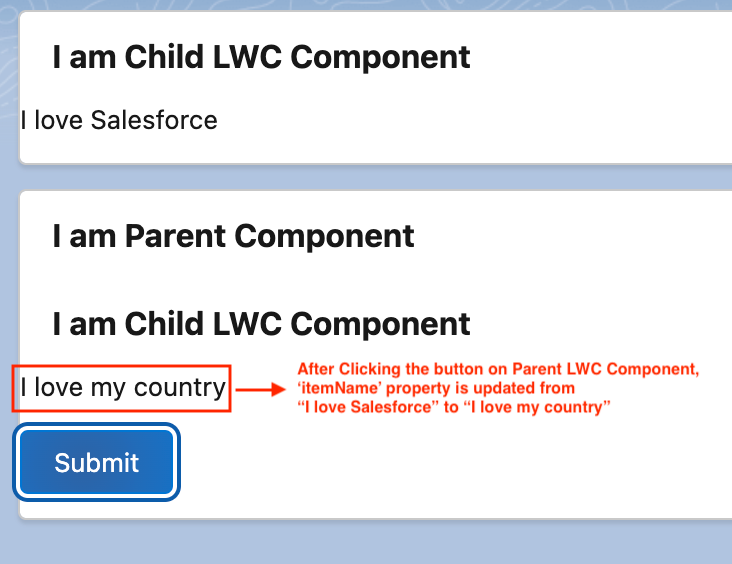
Explanation: How the above Code Works Together
1. Child Component Initialization:
When the child component is loaded, the itemName property is initialized to “I love Salesforce”. This value is displayed in the child component’s UI.
2.Parent Component Button Click:
When the button in the parent component is clicked, the handleClick method in the parent component is executed.
This method locates the child component using this.template.querySelector() and calls the handleChangeValue method in the child component.
3.Updating the Child Component:
The handleChangeValue method in the child component updates the value of the itemName property to “I love my country”.
Since itemName is bound to the child component’s UI, the updated value is immediately reflected.
Key Points to Remember
1. Default Behavior:
@api properties are reactive. So, changes in their values automatically reflect in your UI.
2. Immutable in Child Component:
Public properties that are exposed using @api are read-only in the child component. Modifications of @api properties in the child component throw an error.
3. Performance:
Use @api judiciously for efficient component design. Overexposing properties/methods can lead to tightly coupled components, reducing reusability.
4. Dynamic Interaction:
The parent component can trigger changes in the child component dynamically by invoking its public method.
5. Using querySelector:
‘this.template.querySelector()’ is used to locate the child component in the DOM and call its methods.
Common Use Cases for @api
1. Dynamic Configuration of Child Components:
Expose configuration options via @api properties.
2. Triggering Specific Actions in Child Components:
Allow the parent to control child behaviour using @api methods.
3. Reusable Widgets:
Build versatile components like modals, carousels, or data tables.
Checkout below posts to explore other 2 LWC Decorators in Salesforce
Conclusion
The @api decorator is a powerful tool in Salesforce LWC that facilitates effective component interaction and data sharing.Â
Keep experimenting with different use cases to explore its potential fully.
Happy coding! 🚀
If you have any questions or examples you’d like to share, feel free to comment below! For more insightful posts, visit Writtee.com.
For more details, check out the official documentation: LWC @api Decorator Reference